NSubstitute: simples, sucinto e elegante
NSubstitute é uma framework de simulação em .NET. O NSubstitute cria substitutos de tipos para testes que tanto podem ser mocks (verificação das invocações) como stubs (configuração dos resultados das invocações).
Através de uma sintaxe "fluente", o NSubstitute cria substitutos de tipos para testes que podem ser configurados para retornar resultados específicos das invocações ou podem ser consultados sobre as invocações recebidas.
O NSubstitute está concebido para testes AAA Arrange-Act-Assert.
Exemplo de utilização
Pré-requisitos
- .NET Framework 3.5 ou mais recente
- Adição de Projecto de Testes Unitários à Solução .Net
- Instalação do NSubstitute através de package NuGet ou transferindo e adicionando uma referência para o ficheiro NSubstitute.dll ao projecto de testes unitários
Pressupostos
Conhecimentos de IoCUtilização
Adicionar à classe de testes:using NSubstitute;
Testar a Interface
[TestClass]
public class BusStopAppServiceTests
{
private readonly IBusStopAppService _busStopAppService;
public BusStopAppServiceTests()
{
//Fake repository
var busstops = new List<BusStop>
{
new BusStop {Name = "LIVERPOOL"},
new BusStop {Name = "LIVERPOOL LIME STREET"},
new BusStop {Name = "PADDINGTON"},
new BusStop {Name = "DARTFORD"},
new BusStop {Name = "DARTMOUTH"},
new BusStop {Name = "TOWER HILL"},
new BusStop {Name = "DERBY"}
};
//Create the fake bus stop repository
var busRepository = Substitute.For<IBusStopRepository>);
var cacheManager = Substitute.For<ICacheManager>();
//Arrange
busRepository.GetAllList().Returns(busstops);
//Create AppService by providing the fake repository
_busStopAppService = new BusStopAppService(busRepository, cacheManager);
}
[TestMethod]
public void Return_next_characters_when_busstop_known()
{
//Arrange
var _lookup = "DART";
var expectedCharacterArray = new string[] {"f", "m" };
//Act
var result = _busStopAppService.GetBusStopList(_lookup);
//Assert
CollectionAssert.AreEqual(result.Select(t => t.NextCharacter).Distinct().ToArray(), expectedCharacterArray);
}
[TestMethod]
public void Return_matched_busstops_when_busstop_known()
{
//Arrange
var _lookup = "LIVERPOOL";
var expectedListCount = 2;
//Act
var result = _busStopAppService.GetBusStopList(_lookup);
//Assert
Assert.IsTrue(result.Count().Equals(expectedListCount));
}
[TestMethod]
public void Return_next_empty_character_when_busstop_known()
{
//Arrange
var _lookup = "TOWER";
var expectedCharacter = " ";
//Act
var result = _busStopAppService.GetBusStopList(_lookup);
//Assert
Assert.AreEqual(result.FirstOrDefault().NextCharacter, expectedCharacter);
}
[TestMethod]
public void Return_no_results_when_search_busstop_unknown()
{
//Arrange
var _lookup = "KINGS CROSS";
//Act
var result = _busStopAppService.GetBusStopList(_lookup);
//Assert
Assert.IsTrue(result.Count().Equals(0));
}
}
Resultado
Outras frameworks de substituição (mocking frameworks) para .NET
- Rhino Mocks
- moq
- Simple Mocking
- Microsoft Fakes
Referência: Unit Testing with NSubstitute
Licença CC BY-SA 4.0
Silvia Pinhão Lopes, 29.6.17
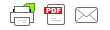
Sem comentários: