Estender os metadados usando atributos de classe em C#
É apresentado como exemplo a aplicação de atributos de classe customizados para obtenção de operação CRUD de um serviço RESTful em tempo de execução.
Declarar a classe Attribute
public class CrudOperationAttribute: Attribute
{
public CrudOperationAttribute() { }
public CrudOperationAttribute(string Create, string Delete, string Retrieve, string RetrieveAll, string Update)
{
this.Create = Create;
this.Retrieve = Retrieve;
this.Delete = Delete;
this.RetrieveAll = RetrieveAll;
this.Update = Update;
}
public string RetrieveAll { get; set; }
public string Retrieve { get; set; }
public string Create { get; set; }
public string Update { get; set; }
public string Delete { get; set; }
}
- A classe é public
- O nome da classe termina com a palavra Attribute por convenção
- A classe herda da classe System.Attribute
Aplicar o atributo de classe
[CrudOperation("createCountry", "deleteCountry/{id}", "retrieveCountry/{id}", "retrieveCountry/{pageNumber}/{recordCount}", "updateCountry/{id}")]
public class Pais
{
public string Nome { get; set; }
public string CodigoPais { get; set; }
}
Obter dinamicamente os parâmetros do atributo de classe como propriedades
public static class RESTfulServiceOperation<T>
{
private static CrudOperationAttribute _crudOperationAttribute = (CrudOperationAttribute)typeof(T).GetCustomAttributes(true)[0];
public static string RetrieveAll { get { return _crudOperationAttribute.RetrieveAll; } }
public static string Retrieve { get { return _crudOperationAttribute.Retrieve; } }
public static string Create { get { return _crudOperationAttribute.Create; } }
public static string Update { get { return _crudOperationAttribute.Update; } }
public static string Delete { get { return _crudOperationAttribute.Delete; } }
}
Invocação
var _resource = RESTfulServiceOperation<Pais>.Retrieve;
RestRequest request = new RestRequest(_resource, Method.GET);
request.AddUrlSegment("id", id.ToString());
RestClient.Execute(request);
Referências: Writing Custom Attributes, C# Attributes in 5 minutes
Licença CC BY-SA 4.0
Silvia Pinhão Lopes, 24.5.18
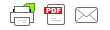
Sem comentários: