Backbone.js sem espinhas
Backbone.js é uma biblioteca MV* de JavaScript com uma interface JSON RESTful e permite preparar as aplicações JS com uma espinha dorsal de Models, Views, Collections e Events resolvendo vários problemas do código esparguete ou spaghetti code.
Exemplo de uma lista de Hellos
index.html
<!DOCTYPE HTML>
<html>
<head>
<title>Hello World Part 2</title>
<meta charset="UTF-8">
</head>
<body>
<div class="wrapper">
<h1>Greetings</h1>
<div id="otherDiv"></div>
<ul id="helloListWithTemplate">
<script type="text/template" id="hello-template">
Hello, <%= name %>
</script>
</ul>
</div>
<!-- JQuery Backbone.js -->
<script src="Scripts/jquery-3.2.1.min.js"></script>
<script src="Scripts/libs/underscore-1.8.3.min.js"></script>
<script src="Scripts/libs/backbone-1.3.3.min.js"></script>
<script src="Scripts/grettings.js"></script>
</body>
</html>
grettings.js (dados locais em memória)
// Backbone.js
$(document).ready(function () {
///
///Model Class Area
///
var Hello = Backbone.Model.extend({
idAttribute: 'id',
defaults: function () {
return {
id: "",
name: "default"
};
}
});
///
///Collection Class Area
///
var HelloList = Backbone.Collection.extend({
model: Hello,
});
///
///View Class Area
///
var HelloViewUsingTemplate = Backbone.View.extend({
tagName: 'li',
model: Hello,
//template: _.template($('#hello-template').html()),
template: $("#hello-template").html(),
render: function () {
//$(this.el).html(this.template(this.model.attributes));
var local_template = _.template(this.template);
this.$el.html(local_template(this.model.attributes));
return this;
}
});
var HelloListViewUsingTemplate = Backbone.View.extend({
el: '#helloListWithTemplate',
initialize: function () {
this.render();
},
render: function () {
this.$el.html();
this.collection.each(function (helloModel) {
var helloViewUsingTemplate = new HelloViewUsingTemplate({
model: helloModel
});
this.$el.append(helloViewUsingTemplate.render().el);
}.bind(this));
return this;
}
});
///
///Model instances Area
///
var helloCollection = new HelloList([{ "id": 1, "name": "Local value 1" }, { "id": 2, "name": "Local value 2" }, { "id": 3, "name": "Local value 3" }], { model: Hello });
//create a new hello collection view instance
var hellolistviewusingtemplate = new HelloListViewUsingTemplate({
collection: helloCollection
});
//JQuery
$('#otherDiv').html('This line does nothing.
');
});
grettings.js (API RESTful)
// Backbone.js
$(document).ready(function () {
///
///Model Class Area
///
var Hello = Backbone.Model.extend({
idAttribute: 'id',
defaults: function () {
return {
id: "",
name: "default"
};
}
});
///
///Collection Class Area
///
var HelloList = Backbone.Collection.extend({
model: Hello,
url: function () { return '/api/Values'; }
});
///
///View Class Area
///
var HelloViewUsingTemplate = Backbone.View.extend({
tagName: 'li',
model: Hello,
//template: _.template($('#hello-template').html()),
template: $("#hello-template").html(),
render: function () {
//$(this.el).html(this.template(this.model.attributes));
var local_template = _.template(this.template);
this.$el.html(local_template(this.model.attributes));
return this;
}
});
var HelloListViewUsingTemplate = Backbone.View.extend({
el: '#helloListWithTemplate',
render: function () {
this.$el.html();
this.collection.each(function (helloModel) {
var helloViewUsingTemplate = new HelloViewUsingTemplate({
model: helloModel
});
this.$el.append(helloViewUsingTemplate.render().el);
}.bind(this));
return this;
}
});
///
///Model instances Area
///
var helloCollection = new HelloList(null, { model: Hello });
helloCollection.reset();
//create a new hello collection view instance
var hellolistviewusingtemplate = new HelloListViewUsingTemplate({
collection: helloCollection
});
helloCollection.fetch({
success: function () {
hellolistviewusingtemplate.render();
}
});
//JQuery
$('#otherDiv').html('This line does nothing.
');
});
Usando a ASP.NET Web API, juntar ao App_Start\WebApiConfig.cs
config.Formatters.JsonFormatter.SerializerSettings.ContractResolver = new CamelCasePropertyNamesContractResolver();
Referência: Introduction to Backbone.js
Licença CC BY-SA 4.0
Silvia Pinhão Lopes, 26.11.17
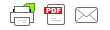
Sem comentários: